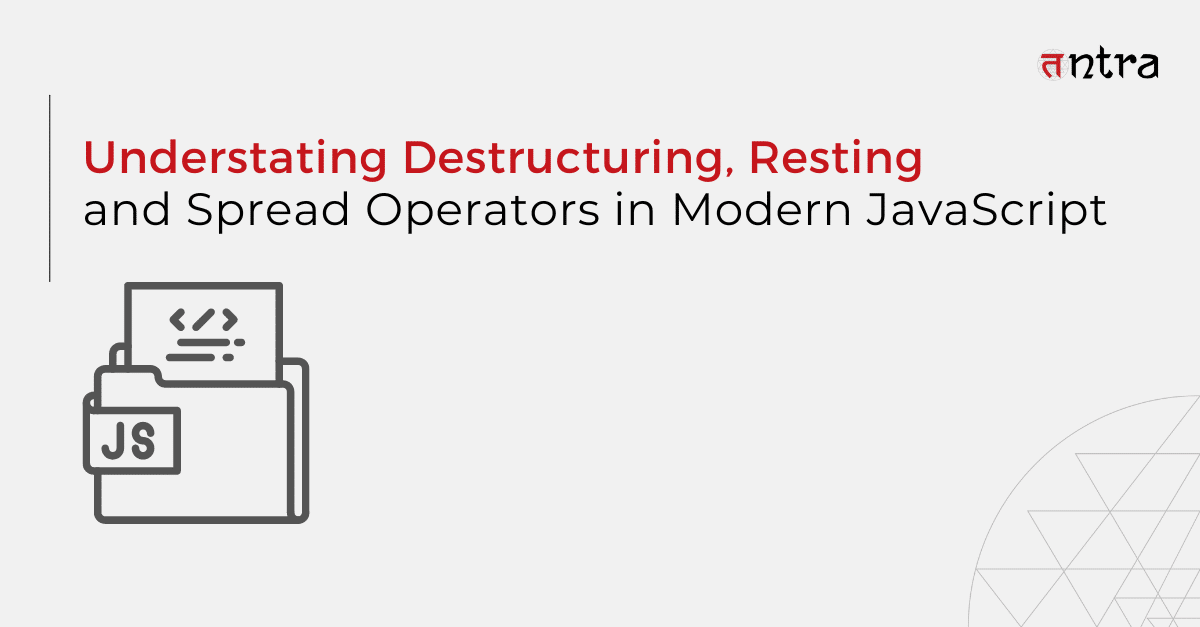
Understating Destructuring, Resting, and Spread Operators in Modern JavaScript
Table of Contents
ToggleWhat is Object Destructuring in JavaScript?
Destructuring array Javascript is an expression that allows us to extract data from arrays, objects, and maps and set them into new, distinct variables. Destructuring object is a feature in JavaScript that will enable you to remove properties from an object and assign them to variables using a shorthand syntax.
It is a way to unpack values from an object and assign them to variables. For example, instead of using the dot notation to access an object’s property, you can use destructuring to assign the property’s value to a variable with the same name as the property.
Syntax:
const { variable1, variable2 } = object;
Example:
const person = { name: 'John Doe', age: 30 };
const { name, age } = person;
console.log(name); // 'John Doe'
console.log(age); // 30
In this example, the properties’ name’ and ‘age’ are being destructured from the object ‘person’ and assigned to the variables’ name’ and ‘age’.
Steps for Object Destructuring in JavaScript
1. Assigning Object value to exist variable
Here’s how to destructure values from an object:
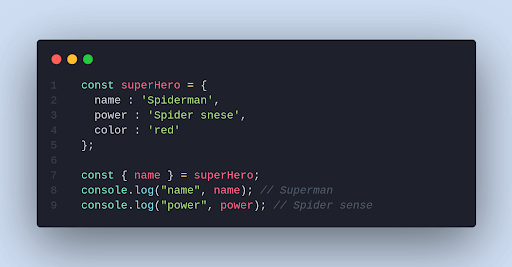
2. Assigning New variable names
The following code is an example of destructuring array of objects into variables with a different name than the object property.
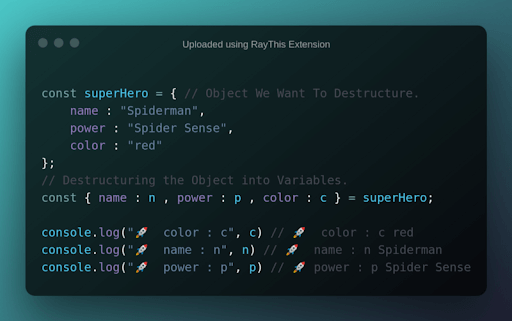
3. Nested Object Destructuring
A nested object is an object that is inside another object. It is a way of organizing and structuring data in which an object contains one or more objects.
These nested objects can also contain other nested objects, creating a hierarchical data structure. This allows for a more complex and flexible way of storing and manipulating data, as properties and methods can be accessed at multiple levels of the nested object.
An object can be nested. This means that the value of an object property can be another object, and so on.
Let’s consider the superHero object below. It has a property called a character with the value of another object. But let’s not stop here! The department has a property with key appearances whose value is another object. Quite a real-life scenario.
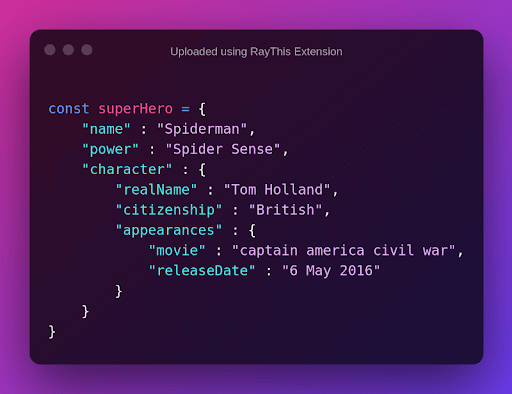
The above screenshot displays how exactly you can destructure an object. Now you have the answer to the question – how object destructuring take place?
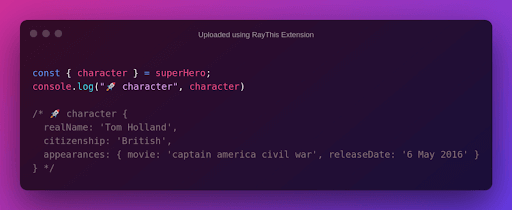
But let’s go one more nested level down. How do we extract the value of the appearance property of the character? Now, this may sound tricky. However, if you apply the same object destructuring principles, you’ll see it’s similar.
Here’s the output when you log appearances:
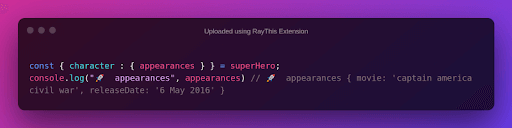
In this case, the character is the key we focus on, and we conduct the array destructuring of its appearance value. Notice the {} around the keys you want to destructure.
Now it’s time to take it to the next level. How do we extract the value of a movie from the character’s movie? We will use the same destructuring object principle again!
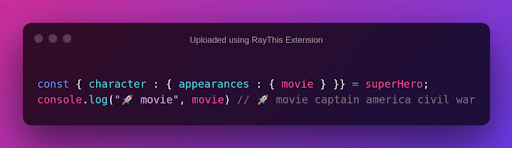
The Output when you log into the movie “civil war”.
Resting and Spread Operator in JavaScript
JavaScript uses three dots (…) for the rest and spread operators. But these two operators are not the same.
1) Spread Operator
The main difference between rest and spread is that the rest operator puts the rest of some specific user-supplied values into a JavaScript array. But the spread syntax expands iterables into individual elements.
2) Rest Operator
A function can be called with any number of arguments, no matter how it is defined.
The Rest parameter syntax (…) instructs the computer to add whatever movies (arguments) supplied by the user into an array. Then, assign that array to the movies parameter.
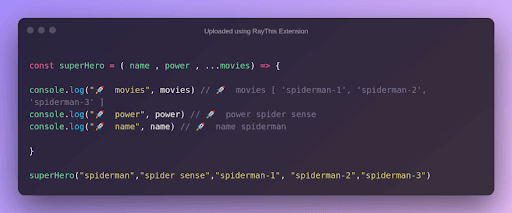
Introduction to Spread Operator in JavaScript
ES6 provides a new operator called spread operator that consists of three dots (…). The spread operator allows you to spread out elements of an iterable object.
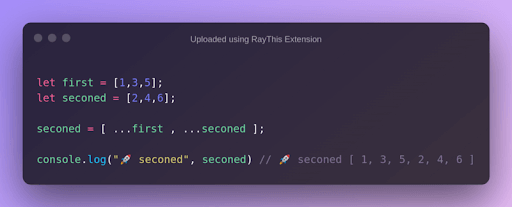
You can shallow-copy objects using (…) spread operators.
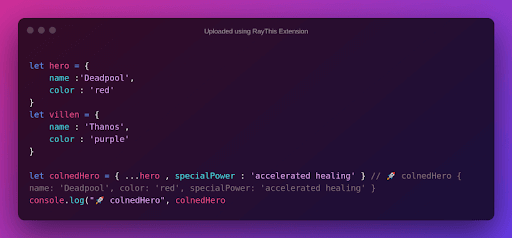
Checkout this cool extension to take code snapshots : https://marketplace.visualstudio.com/items?itemName=Goopware.raythis
In Summary
Object destructuring is a new syntax introduced in ES6, which allows for the creation of variables by extracting an object’s properties in a simplified way. This can be particularly useful when working with frameworks and libraries such as Angular, React, or Vue, where you will be using a lot of syntax for destructuring array of objects.
It’s important to note that object destructuring and spread syntax are not the same things. The spread syntax is used to copy the enumerable properties of an object to create a clone of it and also can be used to update or merge with another object. On the other hand, the rest parameter is used to consolidate or collect the remaining object properties into a new object while destructuring is being done.
For hassle-free Java application development, contact Tntra application development team today.